项目使用Webpack打包,打包完成后生成一些asset,不管是本地开发还是jenkins持续集成,都希望自动上传这些asset到阿里云OSS,于是用nodejs写一个脚本:
1. upload_oss.js
1 | var co = require('co'); |
2. package.json调用
1 | "scripts": { |
项目使用Webpack打包,打包完成后生成一些asset,不管是本地开发还是jenkins持续集成,都希望自动上传这些asset到阿里云OSS,于是用nodejs写一个脚本:
1 | var co = require('co'); |
1 | "scripts": { |
公司前端项目从webpack1.X 升级到webpack2.X,加之技术需求和业务需求增长过快,开发人员没有充足时间深入学习webpack相关技术栈,导致很多配置失效、冗余或者无法辨别究竟有什么用途。
此外,我们基于docker-machine封装了vue、webpack、nodejs等前端开发环境到docker image,docker-machine本身基于Virtual Box,这种虚拟机+Docker的方式又挖了一把大坑。详情见:Docker搭建前端开发环境
综上,几种情况导致我们在docker中使用webpack-dev-server,没办法实时刷新,但在本机开发时却可以。
启动命令为:
VS2017安装Coded UI Test需满足一个大条件:Visual Studio的版本必须是Enterprise版,只有企业版才能安装Coded UI Test。
安装Coded UI Test,需在VS安装界面,进入“单个组件”,勾选“编码的UI测试”,如下图所示:
然而安装完成后却发现新建项目时找不到“编码的UI测试”项目模板:
解决的方法是在安装VS时,在“语言包”界面中勾选一下“英语”,只勾选安装就好,可以不设置VS成英文:
ImageLoadingControl使用说明
控件提供Source
属性,可Binding图片Url或Path
1 | <ImageLoadingControl:ImageLoadingControl HorizontalAlignment="Left" Height="200" Margin="30,68,0,0" VerticalAlignment="Top" Width="200" Source="{Binding ImageUrl1}"/> |
1 | private void button_Click(object sender, RoutedEventArgs e) |
下图分别显示了控件加载中,加载完成,加载失败三种状态:
升级Hexo3.X后发现Landscape-plus主题的分页出现一些问题,由于该主题的GitHub项目也处于长期未维护状态,所以只能自己排查修改。
进入Archives页面后,发现文章列表没有以标题“方块”展示,而是和主页一样以标题和内容展示:
对于这种情况,需要修改Hexo根目录的_config.yml
,加入或修改参数如下所示:
在WPF中进行图片的相关操作是一件比较麻烦的事,并不是说它复杂,而是不注意的话很容易引起内存暴涨甚至溢出。关于BitmapImage使用的相关说明如下:
使用Uri设置BitmapImage会自动形成缓存,不关闭整个模块的话GC不会回收。 故如果在单个模块多次显示图片,不要使用这种方式:
var bitmap = new BitmapImage(new Uri(@"c:\test.bmp"));
建议通过流的方式加载图片:
最近有一个项目需求,要把WPF开发的程序打包成COM组件供其他程序使用,WPF工程转COM并不困难,但有一些细节还是需要记录一下:
首先需要把应用程序转成类库:
需要注意当应用程序转换成类库后App.xaml
就需要删除了,如果在App.xaml
中做了启动控制或者全局资源字典,需要重新规划,如全局资源的加载方式,重复启动的判断等等。
还需要注意要把主窗体改成UserControl,否则组件会以窗口形式打开。
使用EntityFrameWork时,经常会用到lambda表达式来做查询等操作,由于EF要根据表达式生成最终操作数据库的SQL,所以在表达式中加入其它方法如”parse”,”convert”可能会导致不被LinqToEntity识别,异常如下:
System.NotSupportedException: LINQ to Entities does not recognize the method int.Parse(System.String)
但在实际项目中往往会遇到实体字段类型与参数类型需要转换并比较的问题:
例:
自从VS2012去掉了自家的Windows Installer改用InstallSheild之后,打包程序总是找不到满意的工具,最后投奔Advanced Installer,界面简洁,操作简单,功能也很强大。
使用Advanced Installer打包程序的常规步骤如下:
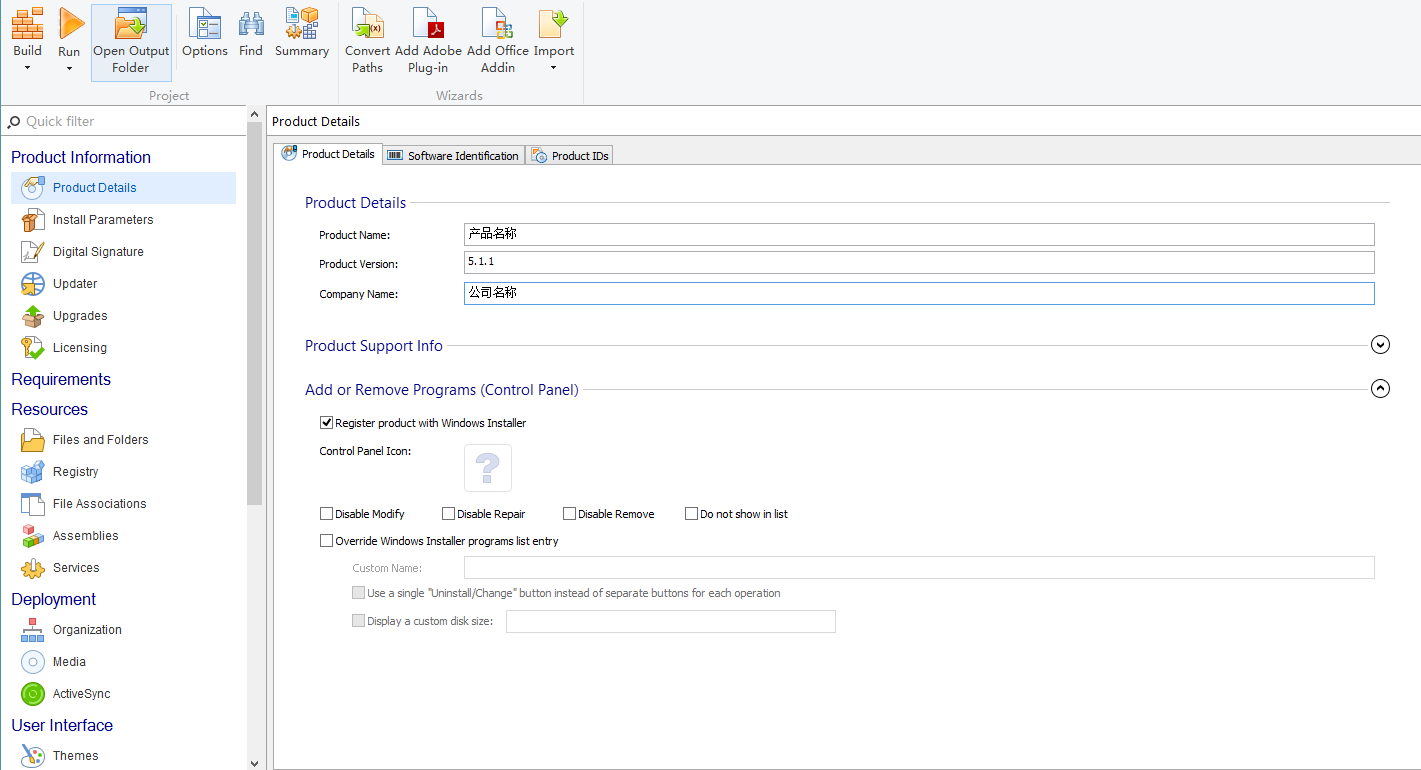
如图所示,填写Product Name, Product Version 和 Product Company. 这里需要解释一下Product Version与Product Code、Update Code之间的关系: